Creating an HTML5 game from scratch can be a challenging but rewarding process. It requires a combination of creative ideas, technical skills, and attention to detail. Here are some key factors to consider when creating an HTML5 game from scratch:
- Game design: This includes deciding on the gameplay mechanics, levels, themes, and other features of your game. You will need to come up with a clear concept for your game and plan out how it will work.
- HTML, CSS, and JavaScript knowledge: HTML is used to structure the content of your game, CSS is used to style the appearance of your game, and JavaScript is used to add interactivity and logic to your game. You will need to have a good understanding of these technologies to create an HTML5 game.
- Development tools: You will need a text editor to write your code and a web browser to test and debug your game. There are many different text editors and web browsers available, and you may want to try out a few to find the ones that work best for you.
- Graphics and sound: Depending on the nature of your game, you may need to create or obtain graphics and sound assets to use in your game. This could include character sprites, backgrounds, sound effects, and music.
- Testing and debugging: It is important to test your game thoroughly as you develop it to ensure it is functioning as intended. You will also need to debug any issues that arise and fix any bugs that are discovered.
- Deployment: Once your game is complete, you will need to decide how to publish and distribute it. This could involve uploading the game files to a web server or hosting platform, or creating a standalone version of the game that can be downloaded and installed on a user’s computer.
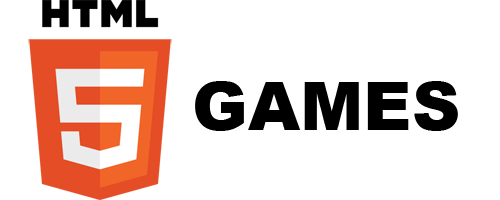
Overall, creating an HTML5 game from scratch requires a combination of creativity, technical skills, and attention to detail. It can be a challenging process, but it can also be very rewarding to see your game come to life and be enjoyed by others.
To create an HTML5 game, you will need to have a basic understanding of HTML, CSS, and JavaScript. Here is a general outline of the steps you can follow:
- Choose a game idea and design the gameplay, levels, and other features.
- Set up your development environment by installing a text editor (such as Sublime Text or Atom) and a web browser (such as Google Chrome or Firefox).
- Create an HTML file for your game and add the necessary HTML elements to structure the content of your game.
- Use CSS to style the appearance of your game, including the layout, colors, and fonts.
- Use JavaScript to add interactivity to your game, such as handling user input and updating the game state. You may also want to consider using a game engine or framework to help with tasks such as rendering graphics and handling physics.
- Test and debug your game to ensure it is functioning as intended.
- Publish your game by uploading the files to a web server or hosting platform.
This is a very broad outline, and there are many additional details and considerations that will depend on the specific requirements of your game. If you are new to HTML, CSS, and JavaScript, you may want to start by learning the basics and working through some tutorials or exercises before attempting to create a full game. There are also many resources and tutorials available online that can help you learn more about creating HTML5 games.
Here is an example of a simple HTML5 game that displays a character on the screen that can be moved using the arrow keys:
<!DOCTYPE html>
<html>
<head>
<style>
/* Style the game */
body {
background-color: lightblue;
}
.character {
position: absolute;
width: 50px;
height: 50px;
background-color: red;
}
</style>
</head>
<body>
<!-- Create the game content -->
<div id="game">
<div id="character" class="character"></div>
</div>
<script>
// Get references to the character and game elements
var character = document.getElementById("character");
var game = document.getElementById("game");
// Set the initial position of the character
character.style.top = "0px";
character.style.left = "0px";
// Listen for arrow key events
document.addEventListener("keydown", function(event) {
// Get the arrow key that was pressed
var key = event.key;
// Get the current position of the character
var top = parseInt(character.style.top);
var left = parseInt(character.style.left);
// Update the position of the character based on the arrow key pressed
if (key == "ArrowUp") {
character.style.top = (top - 10) + "px";
} else if (key == "ArrowDown") {
character.style.top = (top + 10) + "px";
} else if (key == "ArrowLeft") {
character.style.left = (left - 10) + "px";
} else if (key == "ArrowRight") {
character.style.left = (left + 10) + "px";
}
});
</script>
</body>
</html>
This code creates an HTML page with a div
element that represents the character, and uses CSS to style the character as a red box. The JavaScript code listens for keydown events and updates the position of the character based on the arrow key that was pressed.
HTML5 education game idea
Keep in mind that this is just a very basic example, and there are many additional features and considerations that you may want to add to your game, such as handling collisions, displaying scores, and so on.
Here is an idea for a simple educational game that teaches children basic math skills:
Math Racer
In this game, players race against the clock to solve a series of math problems. The game displays a math problem at the top of the screen, and the player has to enter the correct answer using the keyboard. If the player enters the correct answer, the game displays a new problem. If the player enters the wrong answer, the game displays an error message and allows the player to try again.
The game keeps track of the player’s score, and the player earns points for each correct answer. The game also includes a timer that counts down from a set amount of time, and the player has to solve as many problems as possible before the timer runs out.
To make the game more challenging, you could increase the difficulty of the math problems as the player progresses through the game, or you could add power-ups that give the player extra time or help them solve the problems more quickly.
This is just one idea, and there are many other types of educational games that you could create depending on the subject matter and target audience. You could also consider adding other features to the game, such as sound effects, animations, or a user interface to help make the game more engaging and interactive.
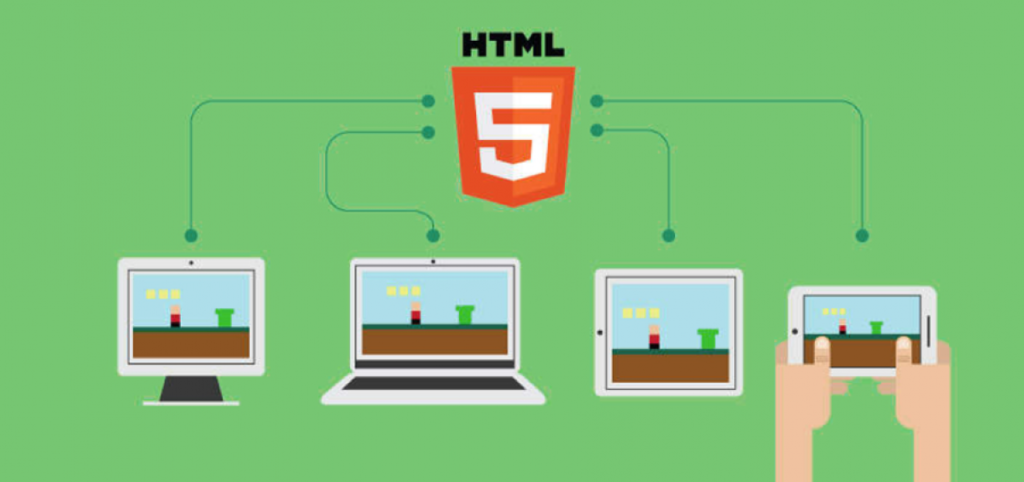
What is an HTML5 game?
An HTML5 game is a type of computer game that is created using HTML5, a markup language used for structuring and presenting content on the World Wide Web. HTML5 games are typically created using a combination of HTML, CSS (Cascading Style Sheets), and JavaScript, and they run in web browsers that support HTML5.
HTML5 games are often created using HTML5 canvas, a feature of HTML5 that allows developers to draw graphics and animations directly in the browser using JavaScript. HTML5 games can be created for a variety of platforms, including desktop computers, mobile devices, and gaming consoles, and they can be played online or offline.
HTML5 games are popular because they are easy to create and distribute, and they can be played on a wide range of devices without the need to install additional software. They are also able to take advantage of new features and technologies that are available in modern web browsers, such as WebGL for 3D graphics and Web Audio for sound.
Overall, HTML5 games are a flexible and widely-supported platform for creating and distributing computer games, and they are used by game developers and players around the world.
HTML5 games can be used in a variety of ways to help people learn, relax, and have fun. Some specific ways in which HTML5 games can help include:
- Education: HTML5 games can be used as educational tools to teach a wide range of subjects, such as math, science, history, and language. Games can be a powerful way to engage students and help them learn new concepts in a fun and interactive way.
- Relaxation and stress relief: HTML5 games can be used to help people relax and de-stress after a long day. Games can provide a way to unwind and take a break from daily life, and they can help people destress and feel more relaxed.
- Socialization: HTML5 games can be used to connect people and foster socialization. Multiplayer games, in particular, can be a great way for people to interact and socialize with others online.
- Exercise the brain: HTML5 games can be used to exercise the brain and improve cognitive skills. Many games, such as puzzle games and strategy games, require players to think critically and problem-solve, which can help improve brain function and cognitive skills.
- Entertainment: HTML5 games can be used to provide entertainment and enjoyment. Games can be a great way to pass the time and have fun, and they can be enjoyed by people of all ages.
Overall, HTML5 games can be a helpful and enjoyable way to learn, relax, socialize, exercise the brain, and have fun.
References:
Wikipedia – https://en.wikipedia.org/wiki/Browser_game
ChatGPT – https://openai.com/blog/chatgpt/
SmartPlay Studios – HTML5 games
Leave a Reply
You must be logged in to post a comment.